A NCommand object can be visualized in three different styles:
Value |
Description |
Image |
The command displays only images.

|
Text |
The command displays text only.

|
ImageAndText |
The command displays both image and text.

|
Default |
The command will let its parent decide in which style to be visualized. The DefaultCommandStyle value of the command parent is used. For example, a command parented by a NMenuWindow will be visualized using ImageAndText style, while the same command parented on a NToolbar will use Image style.
|
The following example demonstrates how to change a command's style (which by default equals to CommandStyle.Default):
C# |
Copy Code
|
NCommand command = new NCommand();
//always display image and text
command.Properties.Style = CommandStyle.ImageAndText;
|
Visual Basic |
Copy Code
|
Dim command As NCommand = New NCommand()
'always display image and text
command.Properties.Style = CommandStyle.ImageAndText
|
A NCommand has native support for displaying images from an image list. It has both image list and image index properties.
The following code demonstrates how to assign a valid image to a command:
C# |
Copy Code
|
ImageList imageList = new ImageList();
//assume that we have a valid bitmap
imageList.Images.AddStrip(myBitmap);
//create a command object and assign it valid image
NCommand command = new NCommand();
command.Properties.ImageList = imageList;
command.Properties.ImageIndex = 0;
|
Visual Basic |
Copy Code
|
Dim imageList As ImageList = New ImageList()
'assume that we have a valid bitmap
imageList.Images.AddStrip(myBitmap)
'create a command object and assign it valid image
Dim command As NCommand = New NCommand()
command.Properties.ImageList = imageList
command.Properties.ImageIndex = 0
|
A command state can change due to either user or framework action.
The following table describes available command states:
Value |
Description |
Default |
The command is in default state.

|
Hovered |
The command is currently under the mouse cursor.

|
Pushed |
The left mouse button is pressed within the command's bounds.

|
DroppedDown |
The command is currently displaying a drop-down menu.
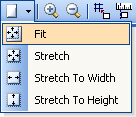
|
A command can give additional feedback in application's context - it might be Checked, Enabled or disabled. By default a command is enabled and unchecked. You may also specify command's visibility using its Visible property - if it is false the command will still exist in its parent collection but will not be displayed.
The following example demonstrates how to use checked/enabled/visible properties:
C# |
Copy Code
|
NCommand command1;
NCommand command2;
NCommand command3;
command1 = new NCommand();
command1.Enabled = false;
command2 = new NCommand();
command2.Checked = true;
command3 = new NCommand();
command3.Properties.Visible = false;
|
Visual Basic |
Copy Code
|
Dim command1 As NCommand
Dim command2 As NCommand
Dim command3 As NCommand
command1 = New NCommand()
command1.Enabled = False
command2 = New NCommand()
command2.Checked = True
command3 = New NCommand()
command3.Properties.Visible = False
|

NCommand object in checked and unchecked state.
For more information about common command properties see
NCommandProperties class.