The image (artistic) frames supported by the background object can greatly increase the visual appearance of the control, because they have an associated fill style, border style, shadow style and shading effect combined with full control over the frame dimensions and style. All this makes this type of frame very flexible and visually attractive.
The properties controlling the appearance of the image border are wrapped in an instance of the NImageFrameStyle class. To better understand the different elements of the image frame lets take a look at the following picture showing the different image frame elements:
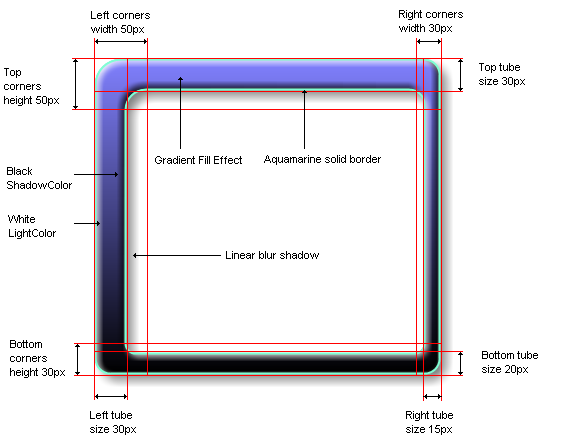
This image shows a rounded image frame with applied horizontal gradient filling, aquamarine border, linear blur shadow and modified settings for corner and tube size. Before we start to elaborate on the different properties of the image frame lets first take a look at the most important property, which is of course the Type property defining the different image frame styles:
Frame type |
Description |
Preview |
None |
No image frame is displayed. |
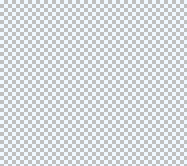 |
Raised |
Image frame with rounded corners that
has no filling only a shading effect
simulating a raised border. |
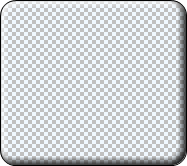 |
Sunken |
Image frame with rounded corners that
has no filling only a shading effect
simulating a sunken border. |
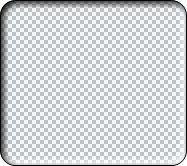 |
Emboss |
Image frame with rounded corners
that completely obscures the background
filling with a shading effect simulating
a raised border. |
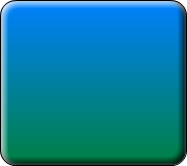 |
Embed |
Image frame with rounded corners
that completely obscures the
background filling with a shading
effect simulating a raised border. |
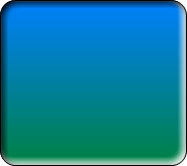 |
Rounded |
Image frame with rounded corners
and filling with controllable corner
and tube sizes. |
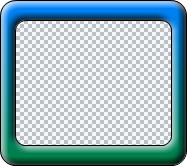 |
RoundedTop |
Image frame with top rounded
corners and square bottom corners. |
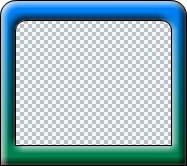 |
RoundedBottom |
Image frame with square top
corners and rounded bottom corners. |
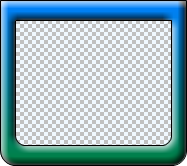 |
RoundedOpenR |
Image frame with rounded corners
and no right tube. |
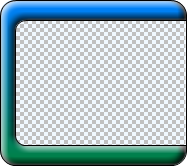 |
RoundedOpenL |
Image frame with rounded corners
and no left tube. |
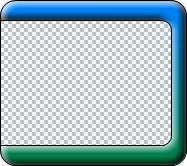 |
RoundedOpenRL |
Image frame with rounded corners
and no left and right tubes. |
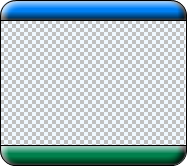 |
Colonial |
Currenty same as RoundedOpenRL |
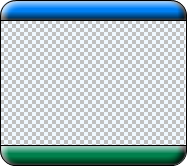 |
Rectangle |
Image frame with square top and
bottom corners. |
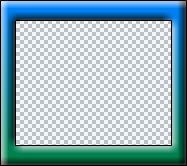 |
RectangleInnerRounded |
Image frame with square outer
corners and rounded inner corners. |
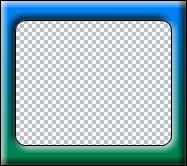 |
RectangleOuterRounded |
Image frame with rounded outer
corners and square inner borders. |
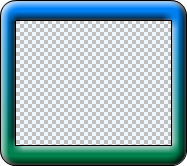 |
Now lets take a closer look at the rest of the properties of the NImageFrameStyle object:
Fill Style
The FillStyle returns a reference to a NFillStyle object controlling the filling applied on the image frame. The following code creates a new image frame style and modifies the fill style applied on it:
C# |
Copy Code
|
NImageFrameStyle imageFrameStyle = new NImageFrameStyle();
imageFrameStyle.FillStyle = new NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant2, Color.AliceBlue, Color.BlanchedAlmond);
|
Visual Basic |
Copy Code
|
Dim imageImageFrameStyle As NImageFrameStyle = New NImageFrameStyle()
imageImageFrameStyle.FillStyle = New NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant2, Color.AliceBlue, Color.BlanchedAlmond)
|
The default fill style applied on the border is white.
Border Style
The BorderStyle property returns a reference to a NStrokeStyle object controlling the border (outline) of the image frame. The following code changes the border color and width:
C# |
Copy Code
|
NImageFrameStyle imageFrameStyle = new NImageFrameStyle();
imageFrameStyle.BorderStyle.Width = new NLength(2, NGraphicsUnit.Pixel);
imageFrameStyle.BorderStyle.Color = Color.Blue;
|
Visual Basic |
Copy Code
|
Dim imageImageFrameStyle As NImageFrameStyle = New NImageFrameStyle()
imageImageFrameStyle.BorderStyle.Width = New NLength(2, NGraphicsUnit.Pixel)
imageImageFrameStyle.BorderStyle.Color = Color.Blue
|
Shadow Style
The image frame style also has an associated shadow, which can be accessed from the ShadowStyleproperty. The following code applies a linear blur shadow with semi transparent black color:
C# |
Copy Code
|
NImageFrameStyle imageFrameStyle = new NImageFrameStyle();
imageFrameStyle.ShadowStyle.Type = ShadowType.LinearBlur;
imageFrameStyle.ShadowStyle.Color = Color.FromArgb(125, 0, 0, 0);
|
Visual Basic |
Copy Code
|
Dim imageImageFrameStyle As NImageFrameStyle = New NImageFrameStyle()
imageImageFrameStyle.ShadowStyle.Type = ShadowType.LinearBlur
imageImageFrameStyle.ShadowStyle.Color = Color.FromArgb(125, 0, 0, 0)
|
Shading Effect
You have probably noticed that the image frame has a shading effect applied on the border edges (look at the the images above). This effect is controlled by the LightColor , ShadowColor and LightEffectSize properties. The following code modifies the settings for these properties:
C# |
Copy Code
|
NImageFrameStyle imageFrameStyle = new NImageFrameStyle();
imageFrameStyle.LightColor = Color.White;
imageFrameStyle.ShadowColor = Color.Navy;
imageFrameStyle.LightEffectSize = new NLength(7, NGraphicsUnit.Pixel);
|
Visual Basic |
Copy Code
|
Dim imageFrameStyle As NImageFrameStyle = New NImageFrameStyle()
imageFrameStyle.LightColor = Color.White
imageFrameStyle.ShadowColor = Color.Navy
imageFrameStyle.LightEffectSize = new NLength(7, NGraphicsUnit.Pixel)
|
Tube and Corner Margins
Every image frame has four tubes and four corners - left, top, right and bottom and their size is controlled via the TubeMargins and CornerMargins properties respectively. Note that the tube size cannot exceed the size of the corner for the corresponding tube (for example the left tube width cannot exceed the size left corner width). If it does the control will automatically clamp it. As with all lengths in the Nevron Graphics tube and corner sizes are specified in NLength format. The following example increases the left tube size:
C# |
Copy Code
|
NImageFrameStyle imageFrameStyle = new NImageFrameStyle();
imageFrameStyle.TubeMargins = New NMarginsL(New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel));
imageFrameStyle.CornerMargins = New NMarginsL(New NLength(40, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel));
|
Visual Basic |
Copy Code
|
Dim imageFrameStyle As NImageFrameStyle = New NImageFrameStyle
imageFrameStyle.TubeMargins = New NMarginsL(New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel))
imageFrameStyle.CornerMargins = New NMarginsL(New NLength(40, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel), New NLength(30, NGraphicsUnit.Pixel))
|
Note that certain frame styles will discard the settings for tube size. For example the RoundedOpenL style discards the left tube size specified by the TubeMargins.Left property.
Round Edge Control
The InnerEdgePercent and the OuterEdgePercent properties control the rounding of the inner and outer edges of the image frame respectively.
The OuterEdgePercent specifies the size of the arc in percents of the frame corner width or height depending on which one of them is smaller.
The InnerEdgePercent specifies the size of the arc in percents of the frame corner width minus the vertical tube width or the frame corner height minus the horizontal tube height, depending on which one of them is smaller.
Note that some frame styles discard the settings for edges (for example the Rectangle frame style, which discards both).
Background color
The background color property controls the color applied on the background of the frame. You may wish to change this property to a different color depending on the background of the form or webpage where the component resides. For example most websites use white page color. The following code changes the background color:
C# |
Copy Code
|
imageFrameStyle.BackgroundColor = Color.White;
|
Visual Basic |
Copy Code
|
imageFrameStyle.BackgroundColor = Color.White
|