The lighting image filter is an advanced type of filter that can emulate the lighting effect produced by two types of light sources - Positional and Directional.
You create a lighting image filter by creating an instance of the NLightingImageFilter class and adding it to the Filters collection of the NImageFiltersStyle object:
C# |
Copy Code
|
NLightingImageFilter lightingImageFilter = new NLightingImageFilter();
someFillStyle.ImageFiltersStyle.Add(lightingImageFilter);
|
Visual Basic |
Copy Code
|
Dim lightingImageFilter As NLightingImageFilter = New NLightingImageFilter()
someFillStyle.ImageFiltersStyle.Add(lightingImageFilter)
|
The LightSourceType property controls the type of lighting to emulate. The default value is LightSourceType.Directional, but you can change this to LightSourceType.Positional at any time:
C# |
Copy Code
|
lightingImageFilter.LightSourceType = LightSourceType.Positional;
|
Visual Basic |
Copy Code
|
lightingImageFilter.LightSourceType = LightSourceType.Positional
|
The Position property of the lighting filter controls where the light comes from.
In the case of Directional light source it defines a direction vector. A value of (0, 0, 1) means that the light rays are falling squarely down the surface.
In the case of positional light source it defines the position of the light source relative to the object being lit. A value of (0, 0, 10) means that the light source is in front of the object. Values greater than 0 for the x dimension will move the light source to the right. Values greater than 0 for the y dimension will move the light source to the top.
To control the color of the light emitted from the light source you need to modify the DiffuseColor and SpecularColor properties. The following code for example sets the diffuse color to Yellow and turns off the specular color contribution:
C# |
Copy Code
|
NLightingImageFilter lightingImageFilter = new NLightingImageFilter();
lightingImageFilter.LightSourceType = LightSourceType.Directional;
lightingImageFilter.DiffuseColor = Color.Yellow;
lightingImageFilter.SpecularColor = Color.Black;
someFillStyle.ImageFiltersStyle.Filters.Add(lightingImageFilter);
|
Visual Basic |
Copy Code
|
Dim lightingImageFilter As NLightingImageFilter = New NLightingImageFilter()
lightingImageFilter.LightSourceType = LightSourceType.Directional
lightingImageFilter.DiffuseColor = Color.Yellow
lightingImageFilter.SpecularColor = Color.Black
someFillStyle.ImageFiltersStyle.Filters.Add(lightingImageFilter)
|
At this point you may be wondering how the control is able to create a surface to lit when the object is specified in 2D. The answer is that it uses the alpha channel of the image to assign different Z values to each pixel. To smoothen this you might consider using the BlurType and BevelDepth properties that control the type of blur to use on the alpha channel before applying the lighting filter to the image:
C# |
Copy Code
|
NLightingImageFilter lightingImageFilter = new NLightingImageFilter();
lightingImageFilter.LightSourceType = LightSourceType.Directional;
lightingImageFilter.DiffuseColor = Color.Yellow;
lightingImageFilter.SpecularColor = Color.Black;
lightingImageFilter.BevelDepth = new NLength(10, NGraphicsUnit.Pixel);
lightingImageFilter.BlurType = BlurType.Linear;
someFillStyle.ImageFiltersStyle.Filters.Add(lightingImageFilter);
|
Visual Basic |
Copy Code
|
Dim lightingImageFilter As NLightingImageFilter = New NLightingImageFilter()
lightingImageFilter.LightSourceType = LightSourceType.Directional
lightingImageFilter.DiffuseColor = Color.Yellow
lightingImageFilter.SpecularColor = Color.Black
lightingImageFilter.BevelDepth = New NLength(10, NGraphicsUnit.Pixel)
lightingImageFilter.BlurType = BlurType.Linear
someFillStyle.ImageFiltersStyle.Filters.Add(lightingImageFilter)
|
Finally lets take a look at a few screenshots showing how the lighting filter works in practice:
Image |
Description |
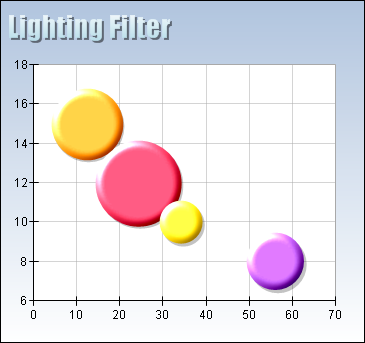 |
Directional Lighting Image Filter |
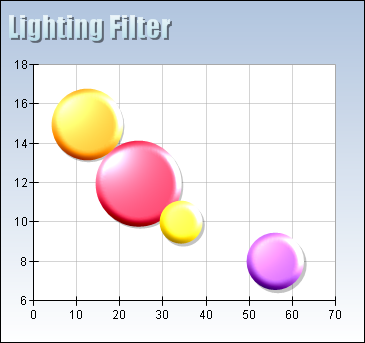 |
Positional Lighting Image Filter |