Table Port Distribution Modes
Table shapes as all other shapes use ports to connect to other shapes, but because table shapes are more complex and can hold more data than a simple shape the question about the proper port distribution arises. In order to cover all scenarios, Nevron Diagram provides you 3 ways of table shape port distribution:
- ShapeBased - treats the table shape as a rectangle and adds a port to each side
- GridBased - treats the table shape as a set of row and columns and adds a port at the beginning and the end of each row and column
- CellBased - treats the table shape as a set of table cells and adds a port to the outer sides of each cell
The following picture illustrates the effect of the different port distribution modes:
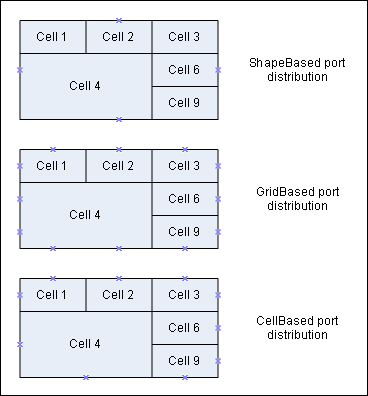
The above diagram can be created using the following code fragments:
Port Distribution Modes Example |
Copy Code
|
private NTableShape CreateTableShape(TablePortDistributionMode portDistributionMode)
{
NTableShape tableShape = new NTableShape(400, 400, 100, 100);
tableShape.PortDistributionMode = portDistributionMode;
tableShape.CellPadding = new Nevron.Diagram.NMargins(10);
tableShape.InitTable(3, 3);
tableShape.BeginUpdate();
for (int i = 0, rowCount = tableShape.RowCount; i < rowCount; i++)
{
for (int j = 0, columnCount = tableShape.ColumnCount; j < columnCount; j++)
{
tableShape[j, i].Text = String.Format("Cell {0}", i * columnCount + j + 1);
}
}
tableShape[0, 1].ColumnSpan = 2;
tableShape[0, 1].RowSpan = 2;
tableShape.EndUpdate();
return tableShape;
}
private void InitDocument(NDrawingDocument document)
{
Array modes = Enum.GetValues(typeof(TablePortDistributionMode));
for (int i = 0; i < modes.Length; i++)
{
TablePortDistributionMode mode = (TablePortDistributionMode)modes.GetValue(i);
NTableShape tableShape = CreateTableShape(mode);
document.ActiveLayer.AddChild(tableShape);
document.ActiveLayer.AddChild(new NTextShape(mode.ToString() + " port distribution", 0, 0, 100, 30));
}
NTableLayout tableLayout = new NTableLayout();
tableLayout.Direction = LayoutDirection.LeftToRight;
tableLayout.ConstrainMode = CellConstrainMode.Ordinal;
tableLayout.MaxOrdinal = 2;
tableLayout.HorizontalSpacing = 30;
tableLayout.VerticalSpacing = 30;
NNodeList shapes = document.ActiveLayer.Children(NFilters.Shape2D);
tableLayout.Layout(shapes, new NDrawingLayoutContext(document));
document.SizeToContent();
}
|