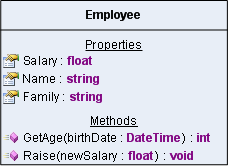
The UML Shape extends the Table Shape in order to provide the needed functionality for easy and good looking visualization of UML class diagrams. You can easily construct an UML shape from a given type by using the InitFromType method (you can import even a whole class hierarchy by using the NClassImporter class and specifying the base class of the hierarchy to its Import method).
To specify whether the class is abstract or not use the Abstract property of the UML shape. You can add members to an UML shape using its Properties and Methods collections. Each method has an Arguments collection that holds its arguments. For each member you can specify whether it is abstract or static and what is its visibility (public, protected, private or package). Abstract members are rendered in italic and static ones are drawn underlined. You can set the preferred style for the visualization of the UML shape by using its MemberFormatStyle Property. The possible values are:
- None- only the names of the members are shown.
- CSharp- the visibility, the type, the names and the arguments (if any) of the members are shown in C# notation.
- CSharp_Short - identical to CSharp, but the visibility of the members is not shown.
- Pascal- the visibility, the type, the names and the arguments (if any) of the members are shown in Pascal notation.
- Pascal_Short- identical to Pascal, but the visibility of the members is not shown.
- Custom- custom notation is used, you must set the MemberFormatString property. Use the constants {VISIBILITY}, {TYPE}, {NAME} and {ARGUMENTS} to create your custom defined format. For example if you want to display a method in C# style use the following format string: <font color='blue'>{VISIBILITY}{TYPE}</font> {NAME}{ARGUMENTS}
Let’s see how easy it is to create the shape presented in the previous chapter:
- Create an instance of the NUmlShape class and add it to the drawing document:
C# |
Copy Code
|
NUmlShape shape = new NUmlShape();
document.ActiveLayer.AddChild(shape);
|
Visual Basic |
Copy Code
|
Dim shape As NUmlShape = New NUmlShape()
document.ActiveLayer.AddChild(shape)
|
- Start the table shape update process by calling the BeginUpdate method and set its name.
Important: always call this method when you need to change more than one property of the table and its cells because it will suppress the auto sizing of the table until the EndUpdate method is called and thus you will experience a significant performance gain:
C# |
Copy Code
|
shape.BeginUpdate();
shape.Name = "Employee";
|
Visual Basic |
Copy Code
|
shape.BeginUpdate()
shape.Name = "Employee"
|
- Add the properties:
C# |
Copy Code
|
shape.Properties.AddChild(new NUmlProperty(MemberVisibility.Public, "float", "Salary", false, false));
shape.Properties.AddChild(new NUmlProperty(MemberVisibility.Public, "string", "Name", false, false));
shape.Properties.AddChild(new NUmlProperty(MemberVisibility.Public, "string", "Family", false, false));
|
Visual Baisc |
Copy Code
|
shape.Properties.AddChild(New NUmlProperty(MemberVisibility.Public, "float", "Salary", False, False))
shape.Properties.AddChild(New NUmlProperty(MemberVisibility.Public, "string", "Name", False, False))
shape.Properties.AddChild(New NUmlProperty(MemberVisibility.Public, "string", "Family", False, False))
|
- Create and add the methods:
C# |
Copy Code
|
NUmlMethod method1 = new NUmlMethod(MemberVisibility.Public, "int", "GetAge", false, false);
shape.Methods.AddChild(method1);
method1.Arguments.AddChild(new NUmlField("DateTime", "birthDate"));
NUmlMethodmethod2 = new NUmlMethod(MemberVisibility.Public, "void", "Raise", false, false);
shape.Methods.AddChild(method2);
method2.Arguments.AddChild(new NUmlField("float", "newSalary"));
|
Visual Basic |
Copy Code
|
Dim method1 As NUmlMethod = New NUmlMethod(MemberVisibility.Public, "int", "GetAge", False, False)
shape.Methods.AddChild(method1)
method1.Arguments.AddChild(New NUmlField("DateTime", "birthDate"))
Dim method2 As NUmlMethod = New NUmlMethod(MemberVisibility.Public, "void", "Raise", False, False)
shape.Methods.AddChild(method2)
method2.Arguments.AddChild(New NUmlField("float", "newSalary"))
|
- Set the member format style:
C# |
Copy Code
|
shape.MemberFormatStyle = MemberFormatStyle.Pascal_Short;
|
Visual Basic |
Copy Code
|
shape.MemberFormatStyle = MemberFormatStyle.Pascal_Short
|
- Finally, do not forget to call the EndUpdate method which will resume the UML shape autosizing feature and will also immediately resize the shape to fit its contents:
C# |
Copy Code
|
shape.EndUpdate();
|
Visual Basic |
Copy Code
|
shape.EndUpdate()
|