In This Topic
The Custom Series allows for painting of custom geometry within a 2D chart. It has the abilities of the chart’s custom painting feature and at the same time behaves like the other series, allowing you to specify its depth order and axis ranges. The following image demonstrates one of the countless possible uses of the custom series:
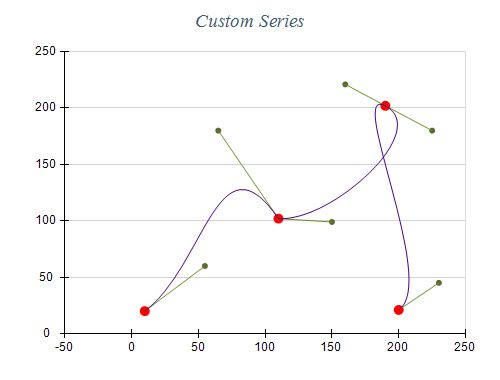
Figure 1.
Creating a custom series
Custom series are represented by the NCustomSeries type. An instance of this type must be added to the series collection of a Cartesian, Polar or Radar chart.
C# |
Copy Code
|
// obtain a reference to the Cartesian chart that is created by default
NCartesianChart chart = (NCartesianChart)chartControl.Charts[0];
// add a custom series
NCustomSeries customSeries = new NCustomSeries();
chart.Series.Add(customSeries);
|
Visual Basic |
Copy Code
|
' obtain a reference to the Cartesian chart that is created by default
Dim chart As NCartesianChart = chartControl.Charts(0)
' add a custom series
Dim customSeries As NCustomSeries = New NCustomSeries
chart.Series.Add(customSeries)
|
Painting
A custom series has no associated data. It displays user painted graphics directly without additional processing. The painting is realized with the help of a callback interface called INCustomSeriesCallback. This interface must be implement by some type, for example:
C# |
Copy Code
|
class MyCustomSeriesCallback : INCustomSeriesCallback
{
public void GetAxisRanges(out NRange1DD rangeX, out NRange1DD rangeY)
{
rangeX = new NRange1DD(0, 10);
rangeY = new NRange1DD(0, 100);
}
public void Paint2D(NChartRenderingContext2D context, NGraphics graphics)
{
// TODO: paint with the graphics object here
}
}
|
Visual Basic |
Copy Code
|
Public Class MyCustomSeries
Implements INCustomSeriesCallback
Public Sub GetAxisRanges(ByRef rangeX As NRange1DD, ByRef rangeY As NRange1DD) Implements _
INCustomSeriesCallback.GetAxisRanges
rangeX = New NRange1DD(0, 10)
rangeY = New NRange1DD(0, 100)
End Sub
Public Sub Paint2D(ByVal context As NChartRenderingContext2D, ByVal graphics As NGraphics) Implements _
INCustomSeriesCallback.Paint2D
' TODO: paint with the graphics object here
End Sub
End Class
|
An instance of the implementing type must be assigned to the Callback property of the NCustomSeries object:
C# |
Copy Code
|
customSeries.Callback = new MyCustomSeriesCallback();
|
Visual Basic |
Copy Code
|
customSeries.Callback = New MyCustomSeriesCallback()
|
The INCustomSeriesCallback interface allows for the series to specify its content range, so that the chart axes are scaled appropriately. For this purpose in the GetAxisRanges method you have to set the actual ranges (in logical coordinates) of the custom series along the X and Y dimensions.
Related Examples
Windows forms: Chart Gallery\Custom\Custom Series
See Also