As with all visual objects in the component the legend has an associated fill style allowing you to control its filling. The following code applies a gradient fill style to the legend:
C# |
Copy Code
|
NLegend legend = chartControl.Legends[0];
legend.FillStyle = new NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.White, Color.Gray);
|
Visual Basic |
Copy Code
|
Dim legend As NLegend = legendsCollection(0)
legend.FillStyle = New NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.White, Color.Gray)
|
The legend grid lines visually separates the data items horizontally and vertically. The following picture shows the different legend grid lines:
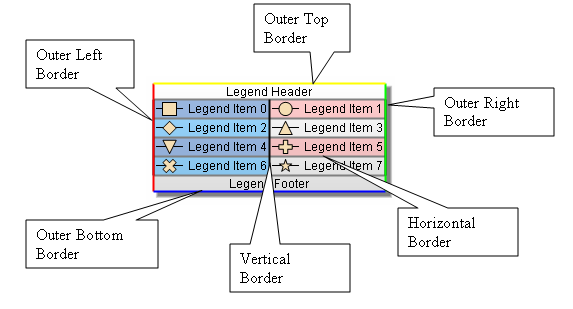
The legend grid lines style is controlled through the HorizontalBorderStyle, VerticalBorderStyle, OuterLeftBorderStyle, OuterTopBorderStyle, OuterRightBorderStyle and OuterBottomBorderStyle properties of the NLegend object. The following code will change the colors of the different grid lines:
C# |
Copy Code
|
NLegend legend = chartControl.Legends[0];
legend.HorizontalBorderStyle.Color = Color.Gray;
legend.VerticalBorderStyle.Color = Color.Black;
legend.OuterLeftBorderStyle.Color = Color.Red;
legend.OuterTopBorderStyle.Color = Color.Yellow;
legend.OuterRightBorderStyle.Color = Color.Green;
legend.OuterBottomBorderStyle.Color = Color.Blue;
|
Visual Basic |
Copy Code
|
Dim legend As NLegend = chartControl.Legends(0)
legend.HorizontalBorderStyle.Color = Color.Gray
legend.VerticalBorderStyle.Color = Color.Black
legend.OuterLeftBorderStyle.Color = Color.Red
legend.OuterTopBorderStyle.Color = Color.Yellow
legend.OuterRightBorderStyle.Color = Color.Green
legend.OuterBottomBorderStyle.Color = Color.Blue
|
The legend also has an associated shadow style controlling the legend shadow. The following code enables the legend shadow and modifies its offset:
C# |
Copy Code
|
NLegend legend = chartControl.Legends[0];
legend.ShadowStyle.Type = ShadowType.GaussianBlur;
legend.ShadowStyle.Offset = new NPointL(5, 5);
|
Visual Basic |
Copy Code
|
Dim legend As NLegend = chartControl.Legends(0)
legend.ShadowStyle.Type = ShadowType.GaussianBlur
legend.ShadowStyle.Offset = New NPointL(5, 5)
|
The legend interlace stripes are automatically generated based on the interlace styles added to the InterlaceStyles collection of the legend. Each interlace style is represented by a NLegendInterlaceStyle object controlling the interlace stripes appearance and orientation (row / column). Furthermore the interlace stripes have Z order - stipes generated by interlace styles on top of the collection appear below interlace stripes generated by the styles afterwards. The following code creates two row interlace styles with gradient filling:
C# |
Copy Code
|
NLegend legend = chartControl.Legends[0];
// switch to manual mode
legend.Mode = LegendMode.Manual;
// feed some data
for (int i = 0; i < 60; i++)
{
NLegendItemCellData ldi = new NLegendItemCellData();
ldi.Text = "Legend Data Item " + i.ToString();
ldi.MarkShape = LegendMarkShape.None;
ldi.MarkLineStyle.Width = new NLength(0);
legend.Data.Items.Add(ldi);
}
// apply expand strategy
legend.Data.ExpandMode = LegendExpandMode.ColsFixed;
legend.Data.ColCount = 4;
// apply row interlacing
NLegendInterlaceStyle rowInterlaceStyle = new NLegendInterlaceStyle();
rowInterlaceStyle.Type = LegendInterlaceStyleType.Row;
rowInterlaceStyle.FillStyle = new NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.SpringGreen, Color.Teal);
rowInterlaceStyle.Interval = 2;
rowInterlaceStyle.Length = 2;
legend.InterlaceStyles.Add(rowInterlaceStyle);
// apply row interlacing
rowInterlaceStyle = new NLegendInterlaceStyle();
rowInterlaceStyle.Type = LegendInterlaceStyleType.Row;
rowInterlaceStyle.FillStyle = new NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.LightBlue, Color.RoyalBlue);
rowInterlaceStyle.Begin = 2;
rowInterlaceStyle.Interval = 2;
rowInterlaceStyle.Length = 2;
legend.InterlaceStyles.Add(rowInterlaceStyle);
|
Visual Basic |
Copy Code
|
Dim legend As NLegend = chartControl.Legends(0)
' switch to manual mode
legend.Mode = LegendMode.Manual
' feed some data
For i As Integer = 0 To 59
Dim ldi As New NLegendItemCellData
ldi.Text = "Legend Data Item " + i.ToString()
ldi.MarkShape = LegendMarkShape.None
ldi.MarkLineStyle.Width = New NLength(0)
legend.Data.Items.Add(ldi)
Next i
' apply expand strategy
legend.Data.ExpandMode = LegendExpandMode.ColsFixed
legend.Data.ColCount = 4
' apply row interlacing
Dim rowInterlaceStyle As New NLegendInterlaceStyle
rowInterlaceStyle.Type = LegendInterlaceStyleType.Row
rowInterlaceStyle.FillStyle = New NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.SpringGreen, Color.Teal)
rowInterlaceStyle.Interval = 2
rowInterlaceStyle.Length = 2
legend.InterlaceStyles.Add(rowInterlaceStyle)
' apply row interlacing
rowInterlaceStyle = New NLegendInterlaceStyle
rowInterlaceStyle.Type = LegendInterlaceStyleType.Row
rowInterlaceStyle.FillStyle = New NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.LightBlue, Color.RoyalBlue)
rowInterlaceStyle.Begin = 2
rowInterlaceStyle.Interval = 2
rowInterlaceStyle.Length = 2
legend.InterlaceStyles.Add(rowInterlaceStyle)
|
This code will produce the following legend appearance:
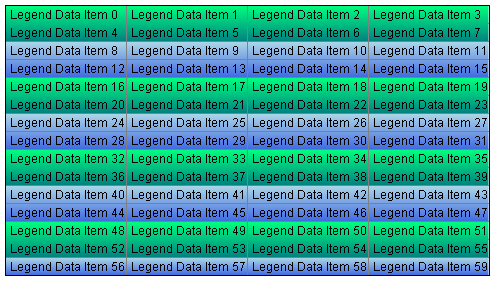
Now lets add a column interlace style with that appears on top of the row interlace styles and highlights the first row with a white to red vertical gradient:
C# |
Copy Code
|
// apply column interlacing
NLegendInterlaceStyle columnInterlaceStyle = new NLegendInterlaceStyle();
columnInterlaceStyle.Type = LegendInterlaceStyleType.Col;
columnInterlaceStyle.FillStyle = new NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.White, Color.Red);
columnInterlaceStyle.Infinite = false;
columnInterlaceStyle.End = 1;
legend.InterlaceStyles.Add(columnInterlaceStyle);
|
Example Title |
Copy Code
|
' apply column interlacing
Dim columnInterlaceStyle As New NLegendInterlaceStyle
columnInterlaceStyle.Type = LegendInterlaceStyleType.Col
columnInterlaceStyle.FillStyle = New NGradientFillStyle(GradientStyle.Horizontal, GradientVariant.Variant1, Color.White, Color.Red)
columnInterlaceStyle.Infinite = False
columnInterlaceStyle.End = 1
legend.InterlaceStyles.Add(columnInterlaceStyle)
|
The legend appearance will now change to:
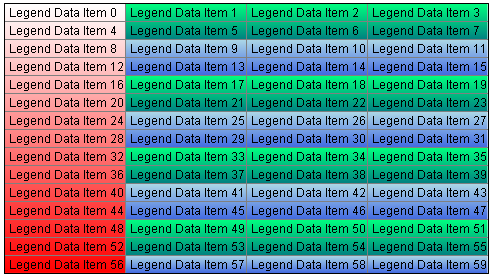
Windows Forms \ Panels \ Legend
Web Forms \ Panels \ Legend